The most important thing when building the web API is creating API documentation. With API documentation, it will be easier for another programmer to integrate their application with your web service.
In this post, I will show how to integrate Go net/http package and swagger using http-swagger package.
Installation
First, we have to install swag package.
$ go get github.com/swaggo/swag/cmd/swag
Initialization
Then we have to generate first `docs` folder, so we could import it to the application. Please run it on root folder of the project.
$ swag init
After generate docs folder, we import the package and generated docs. In this case, my project name is goweb.
import (
httpSwagger "github.com/swaggo/http-swagger"
_ "goweb/docs"
)
We have to initialize the general info for the documentation. It will be placed before main function. It describes the name, description, contact, etc. For the detail, we could see from the swag documentation.
// @title Go Restful API with Swagger
// @version 1.0
// @description Simple swagger implementation in Go HTTP
// @contact.name Linggar Primahastoko
// @contact.url https://linggar.asia
// @contact.email x@linggar.asia
// @securityDefinitions.apikey ApiKeyAuth
// @in header
// @name Authorization
// @host localhost:8082
// @BasePath /
func main() {
...
}
We add these blocks to tell swagger that we will be using token in header for the authentication. So it means, if we want directly access some pages that using authentication, we have to pass Authorization: Basic xxxyyytokenzzzaaa
// @securityDefinitions.apikey ApiKeyAuth
// @in header
// @name Authorization
Implementation
We will write the documentation on each function and placed before the function itself. For the parameter and response, we just have to write the struct name on the documentation because we already have defined the structs. This is the part I like the most, because we don’t have to define the structure again in the documentation part.
type authParam struct {
Username string `json:"username"`
Password string `json:"password"`
}
type authResp struct {
Token string `json:"token"`
}
// authLogin godoc
// @Summary Auth Login
// @Description Auth Login
// @Tags auth
// @ID auth-login
// @Accept json
// @Produce json
// @Param authLogin body main.authParam true "Auth Login Input"
// @Success 200 {object} main.authResp
// @Router /auth/login [post]
func authLogin(w http.ResponseWriter, r *http.Request) {
...
}
That is the login function, so we don’t have to pass the token to access the resource. But what if we want to authorize with token in swagger? Yes, we only have to add // @Security ApiKeyAuth.
// userProfile godoc
// @Summary User Profile
// @Description User Profile
// @Tags users
// @ID user-profile
// @Accept json
// @Produce json
// @Success 200 {object} main.meResp
// @Router /users/profile [get]
// @Security ApiKeyAuth
func userProfile(w http.ResponseWriter, r *http.Request) {
...
}
Then finally we have to add the routing and swagger function in the main function, so we could access it in the browser.
func main() {
http.HandleFunc("/swagger/", httpSwagger.WrapHandler)
...
http.ListenAndServe(":8082", nil)
}
After we setup all the components, we have to generate the documentation with swag command again.
$ swag init
2019/01/29 20:29:34 Generate swagger docs....
2019/01/29 20:29:34 Generate general API Info
2019/01/29 20:29:34 Generating main.authParam
2019/01/29 20:29:34 Generating main.authResp
2019/01/29 20:29:34 Generating main.meResp
2019/01/29 20:29:34 create docs.go at docs/docs.go
Finally, we could build and run the application. We could access the documentation in http://<host>:<port>/swagger/index.html.
Example
This is the full implementation to integrate swagger with Go net/http package. This is only an example. It is not a perfect code that you could just copy and paste into your web app, because actually, we could write with better handler, wrapper, and middleware. For the detail of username, password, and token, you could get that information from the code. So, please enjoy! 😀
main.go
package main
import (
"encoding/json"
httpSwagger "github.com/swaggo/http-swagger"
"log"
"net/http"
"strings"
_ "goweb/docs"
)
type errorResp struct {
Error string `json:"error"`
}
type indexResp struct {
Message string `json:"message"`
}
type meResp struct {
Username string `json:"username"`
Fullname string `json:"fullname"`
}
type authResp struct {
Token string `json:"token"`
}
type authParam struct {
Username string `json:"username"`
Password string `json:"password"`
}
// writeJSON provides function to format output response in JSON
func writeJSON(w http.ResponseWriter, code int, payload interface{}) {
resp, err := json.Marshal(payload)
if err != nil {
log.Println("Error Parsing JSON")
}
w.Header().Set("Content-type", "application/json")
w.WriteHeader(code)
w.Write(resp)
}
// basicAuthMW is middleware function to check whether user is authenticated or not
// actually you could write better code for this function
func basicAuthMW(w http.ResponseWriter, r *http.Request) map[string]string {
errorAuth := errorResp{
Error: "Unauthorized access",
}
header := r.Header.Get("Authorization")
if header == "" {
writeJSON(w, 401, errorAuth)
return map[string]string{}
}
apiKey := strings.Split(header, " ")
if len(apiKey) != 2 {
writeJSON(w, 401, errorAuth)
return map[string]string{}
}
if apiKey[0] != "Basic" {
writeJSON(w, 401, errorAuth)
return map[string]string{}
}
users := map[string]map[string]string{
"28b662d883b6d76fd96e4ddc5e9ba780": map[string]string{
"username": "linggar",
"fullname": "Linggar Primahastoko",
},
}
if _ , ok := users[apiKey[1]]; !ok {
writeJSON(w, 401, errorAuth)
return map[string]string{}
}
return users[apiKey[1]]
}
func decodePost(r *http.Request, structure interface{}) {
decoder := json.NewDecoder(r.Body)
err := decoder.Decode(structure)
if err != nil {
log.Println("Error parsing post data")
}
}
// authLogin godoc
// @Summary Auth Login
// @Description Auth Login
// @Tags auth
// @ID auth-login
// @Accept json
// @Produce json
// @Param authLogin body main.authParam true "Auth Login Input"
// @Success 200 {object} main.authResp
// @Router /auth/login [post]
func authLogin(w http.ResponseWriter, r *http.Request) {
var param authParam
decodePost(r, ¶m)
if param.Username == "linggar" && param.Password == "linggar" {
respAuth := authResp{
Token: "28b662d883b6d76fd96e4ddc5e9ba780",
}
writeJSON(w, 200, respAuth)
} else {
failResp := errorResp{
Error: "Wrong username/password",
}
writeJSON(w, 401, failResp)
}
}
// userProfile godoc
// @Summary User Profile
// @Description User Profile
// @Tags users
// @ID user-profile
// @Accept json
// @Produce json
// @Success 200 {object} main.meResp
// @Router /users/profile [get]
// @Security ApiKeyAuth
func userProfile(w http.ResponseWriter, r *http.Request) {
info := basicAuthMW(w, r)
if len(info) == 0 {
return
}
respMe := meResp{
Username: info["username"],
Fullname: info["fullname"],
}
writeJSON(w, 200, respMe)
}
// @title Go Restful API with Swagger
// @version 1.0
// @description Simple swagger implementation in Go HTTP
// @contact.name Linggar Primahastoko
// @contact.url https://linggar.asia
// @contact.email x@linggar.asia
// @securityDefinitions.apikey ApiKeyAuth
// @in header
// @name Authorization
// @host localhost:8082
// @BasePath /
func main() {
http.HandleFunc("/swagger/", httpSwagger.WrapHandler)
http.HandleFunc("/auth/login", authLogin)
http.HandleFunc("/users/profile", userProfile)
http.ListenAndServe(":8082", nil)
}
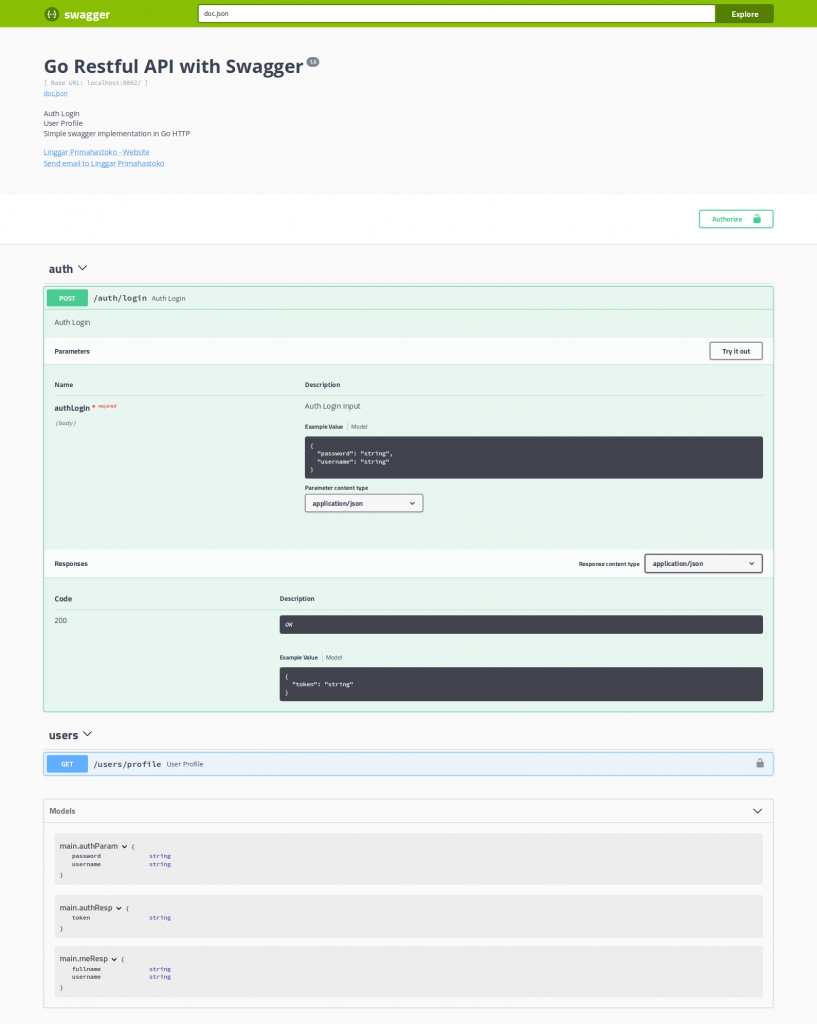