Setelah hampir dua tahun tidak menulis sepatah kata pun di blog ini, akhirnya saya memutuskan untuk sedikit berbagi lagi hal-hal terkait teknologi informasi, tentunya hal-hal yang sedang saya alami dan saya kerjakan. Apa yang akan saya bahas adalah tentang sebuah aplikasi Windows Phone 8.1 yang kemarin baru saja saya install, yaitu Easy Transfer. Easy Transfer adalah aplikasi yang memudahkan penggunanya untuk melakukan manajemen berkas pada sebuah device berbasis Windows melalui browser pada komputer (kebetulan saya memakai Nokia Lumia 920).
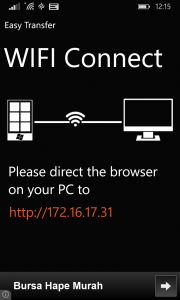
Aplikasi Easy Transfer ini sangat memudahkan saya untuk memindahkan berkas dari handphone saya ke komputer, ataupun sebaliknya melalui wifi dengan protokol HTTP. Sebelumnya saya agak kesulitan dalam memindahkan berkas dari handphone karena laptop saya menggunakan OS Linux dan mtpfs yang digunakan di Linux agak rewel ketika harus berhadapan dengan Nokia Lumia.
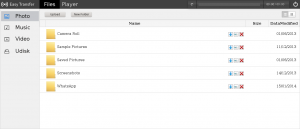
Unduh, unggah, penamaan ulang, dan menghapus berkas dapat dilakukan melalui browser ketika kita sudah menginstall aplikasi Easy Transfer di device Windows Phone yang kita pakai. Tetapi ketika saya menggunakan, ada hal yang kurang pas dalam aplikasi ini, yaitu akses yang dilakukan tanpa menggunakan autentikasi. Jadi, ketika kita mengetahui alamat ip dari sebuah device Windows Phone yang sedang membuka aplikasi Easy Transfer, kita langsung dapat mengaksesnya. Lalu berbekal pemikiran itu, akhirnya saya membuat sebuah script sederhana untuk melakukan scanning dalam jaringan yang sedang saya gunakan terhadap device yang sedang membuka Easy Transfer. 😛
#!/usr/bin/env python
import argparse
import sys
import socket
import fcntl
import struct
import time
import multiprocessing
import urllib2
import re
import datetime
def unwrap_self_f(arg, **kwarg):
return GetEasy.scan_easy(*arg, **kwarg)
class GetEasy:
def __init__(self):
self.now_int = time.time()
def get_self_ip(self, device):
# get ip address code from activestate
# http://code.activestate.com/recipes/439094-get-the-ip-address-associated-with-a-network-inter/
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
try:
my_ip = socket.inet_ntoa(fcntl.ioctl(
s.fileno(),
0x8915, # SIOCGIFADDR
struct.pack('256s', device[:15])
)[20:24])
return my_ip
except:
return False
def scan_easy(self, host):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
result = sock.connect_ex((host, 80))
if result == 0:
try:
get_page = urllib2.urlopen('http://%s' %host).read()
if re.search(r'Easy Transfer', get_page):
print 'Easy Transfer Detected in %s' %host
return host
except Exception, err:
pass
def run(self, threads, ip_range):
pool = multiprocessing.Pool(processes=int(threads))
hosts = []
for i in xrange(1,255):
hosts.append('%s.%d' %('.'.join(ip_range.split('.')[0:3]), i))
get_ips = pool.map(unwrap_self_f, zip([self]*len(hosts), hosts))
return get_ips
if __name__ == '__main__':
now_int = time.time()
parser = argparse.ArgumentParser()
parser.add_argument('-i', '--interface', help='network interface')
parser.add_argument('-t', '--threads', help='total threads, if not specified, will use single thread')
args = parser.parse_args()
if len(sys.argv) == 1:
parser.print_help()
sys.exit(1)
if not args.interface:
parser.print_help()
sys.exit(1)
if not args.threads:
threads = 1
else:
threads = args.threads
c = GetEasy()
ip_addr = c.get_self_ip(args.interface)
if not ip_addr:
print 'invalid interface'
sys.exit(1)
print 'scanning wifi area...'
Detected = c.run(threads, ip_addr)
scanned_time = time.time() - now_int
print '255 hosts scanned in %s' %(datetime.timedelta(seconds=scanned_time))
Lalu ketika dijalankan, hasilnya adalah sebagai berikut
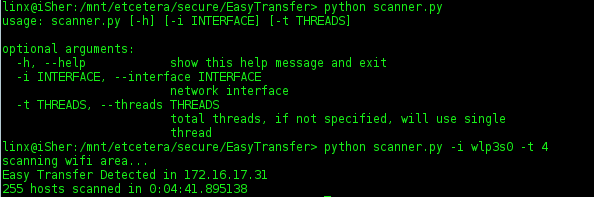
Setelah scanning dilakukan dan terdeteksi ada orang yang membuka aplikasi Easy Transfer, kita bisa mengakses alamat ipnya langsung di browser dan kita bisa melakukan apa saja dengan berkas pada ip itu. 😛
Lalu bagaimana cara kita mengakses berkas dengan menggunakan Easy Transfer secara aman? Caranya gampang, yaitu gunakan fitur internet sharing pada Windows Phone, agar jaringan yang kita gunakan adalah jaringan pribadi, bukan jaringan terbuka yang bisa diakses siapa saja. Tentunya dengan memberikan kata kunci yang agak sulit untuk ditebak juga. Ingat, jangan percaya bahwa semua yang ada di dalam satu jaringan itu adalah orang baik, bisa saja yang terlihat baik itu adalah orang yang sedang mengakses data-data kamu. Kalau saya? Ya orang baiklah 😀